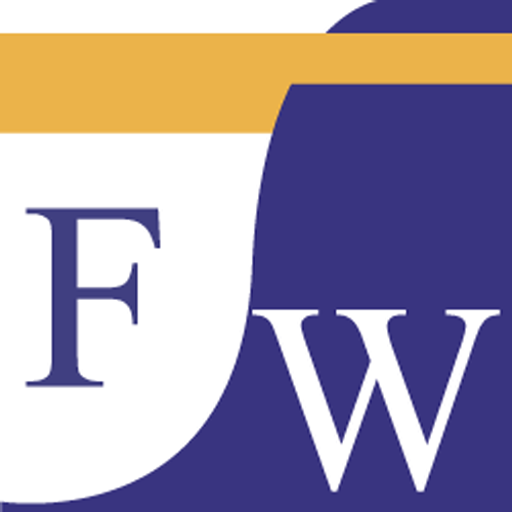
Introduction
Golang library to manage password functions
We use these functions in several projects at Frontware to handle passwords.

Functions
IsCommon
The function retruns trye if the password is in the list of 1 million most used password.
When a user signs up, you can use this function to make sure he is not providing a password that we can easily find in dicitonaries.
The function uses Bloomfilter to keep a low memory consumption.
The list of common passwords comes from https://github.com/danielmiessler/SecLists.
Get the list of million passwords here.
HashPassword
Returns a bcrypt hash of the password as a string.
Never store a password, always store the bcrypt hash to later identify a user.
NewPassword
Returns a new random password.
It's never a good idea to let user set their own password. This functions generates a random password for your user.
Install
If you use module just import github.com/frontware/pwd
in your code.
If you don't use go mod then you download the module:
go get github.com/frontware/pwd
Example
Sample code
package main
import (
"fmt"
"github.com/frontware/pwd"
)
func main() {
// Generate new password of 8 chars
pass := pwd.NewPassword(8)
// Get hash
hash, _ := pwd.HashPassword(pass)
fmt.Println("Password\t\t", pass)
fmt.Println("Hash\t\t\t", hash)
fmt.Println()
fmt.Println()
fmt.Println("Common passwords")
fmt.Println()
fmt.Println("Password fjdslkjflkd\t", pwd.IsCommon("fjdslkjflkd"))
fmt.Println("Password qwerty\t\t", pwd.IsCommon("qwerty"))
}

Results
Password 5EZoCLds
Hash $2a$10$yoijtlT0xosZcTd.XWOLUe04zUiNrJj1TAZZSAxJC1zQX/lL.yrhG
Common password
Password fjdslkjflkd false
Password qwerty true
Doc
See the doc online

© 2020 Frontware International Co,Ltd. All Rights Reserved.