Goodhosts
Simple hosts file (/etc/hosts
) management in Go (golang).

A Surrealist Parisian Dinner Party chez Madame Rothschild, 1972

Features
- List, add, remove and check hosts file entries from code or the command-line.
- Windows support.
Command-Line Usage
Installation
Download the binary and put it in your path.
$ wget https://github.com/lextoumbourou/goodhosts/releases/download/v2.0.0/goodhosts
$ chmod +x goodhosts
$ export PATH=$(pwd):$PATH
$ goodhosts --help
List entries
$ goodhosts list
127.0.0.1 localhost
10.0.0.5 my-home-server xbmc-server
10.0.0.6 my-desktop
Add --all
flag to include comments.
Check for an entry
$ goodhosts check 127.0.0.1 facebook
Add an entry
$ goodhosts add 127.0.0.1 facebook
Or entries.
$ goodhosts add 127.0.0.1 facebook twitter gmail
Remove an entry
$ goodhosts rm 127.0.0.1 facebook
Or entries.
$ goodhosts rm 127.0.0.1 facebook twitter gmail
More
$ goodhosts --help
API Usage
Installation
$ go get github.com/lextoumbourou/goodhosts
List entries
package main
import (
"fmt"
"github.com/lextoumbourou/goodhosts"
)
func main() {
hosts := goodhosts.NewHosts()
for _, line := range hosts.Lines {
fmt.Println(line.Raw)
}
}
Check for an entry
package main
import (
"fmt"
"github.com/lextoumbourou/goodhosts"
)
func main() {
hosts := goodhosts.NewHosts()
if hosts.Has("127.0.0.1", "facebook") {
fmt.Println("Entry exists!")
return
}
fmt.Println("Entry doesn't exist!")
}
Add an entry
package main
import (
"fmt"
"github.com/lextoumbourou/goodhosts"
)
func main() {
hosts := goodhosts.NewHosts()
// Note that nothing will be added to the hosts file until ``hosts.Flush`` is called.
hosts.Add("127.0.0.1", "facebook.com", "twitter")
if err := hosts.Flush(); err != nil {
panic(err)
}
}
Remove an entry
package main
import (
"fmt"
"github.com/lextoumbourou/goodhosts"
)
func main() {
hosts := goodhosts.NewHosts()
// Same deal, yo: call hosts.Flush() to make permanent.
hosts.Remove("127.0.0.1", "facebook", "twitter")
if err := hosts.Flush(); err != nil {
panic(err)
}
}
Changelog
2.0.0 (2015-05-04)
- Breaking API change.
- Add support for adding and removing multiple hosts.
- Added
--all
flag.
- Handle malformed IP addresses.
1.0.0 (2015-05-03)
License
MIT
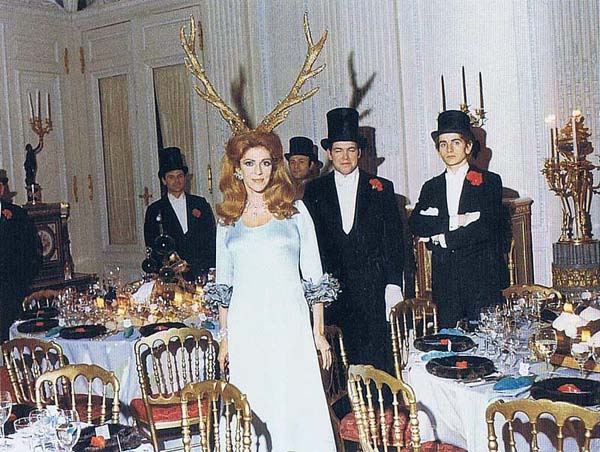