Goodhosts

This is a fork of [https://github.com/lextoumbourou/goodhosts] as we needed a bit deeper management.
Simple hosts file (/etc/hosts
) management in Go (golang).
Features
- List, add, remove and check hosts file entries from code or the command-line.
- Windows support.
Command-Line Usage
List entries
$ goodhosts list
127.0.0.1 localhost
10.0.0.5 my-home-server xbmc-server
10.0.0.6 my-desktop
Add --all
flag to include comments.
Check for an entry
$ goodhosts check 127.0.0.1 facebook.com
Add an entry
$ goodhosts add 127.0.0.1 facebook.com
Or entries.
$ goodhosts add 127.0.0.1 facebook.com twitter.com gmail.com
Remove an entry
$ goodhosts rm 127.0.0.1 facebook.com
Or entries.
$ goodhosts rm 127.0.0.1 facebook.com twitter.com gmail.com
More
$ goodhosts --help
API Usage
Installation
$ go get github.com/ChrisWiegman/goodhosts
List entries
package main
import (
"fmt"
"github.com/ChrisWiegman/goodhosts"
)
func main() {
hosts := goodhosts.NewHosts()
for _, line := range hosts.Lines {
fmt.Println(line.Raw)
}
}
Check for an entry
package main
import (
"fmt"
"github.com/ChrisWiegman/goodhosts"
)
func main() {
hosts := goodhosts.NewHosts()
if hosts.Has("127.0.0.1", "facebook.com") {
fmt.Println("Entry exists!")
return
}
fmt.Println("Entry doesn't exist!")
}
Add an entry
package main
import (
"fmt"
"github.com/ChrisWiegman/goodhosts"
)
func main() {
hosts := goodhosts.NewHosts()
// Note that nothing will be added to the hosts file until ``hosts.Flush`` is called.
hosts.Add("127.0.0.1", "This is a line comment", "facebook.com", "twitter.com")
if err := hosts.Flush(); err != nil {
panic(err)
}
}
Remove an entry
package main
import (
"fmt"
"github.com/ChrisWiegman/goodhosts"
)
func main() {
hosts := goodhosts.NewHosts()
// Same deal, yo: call hosts.Flush() to make permanent.
hosts.Remove("127.0.0.1", "facebook.com", "twitter.com")
if err := hosts.Flush(); err != nil {
panic(err)
}
}
Changelog
3.2 (2019-10-10)
- Add ability to remove an entire section
3.1.1 (2019-10-10)
3.1 (2019-10-10)
- Allow sectioning of IP addresses with "section name" in api
- Various bugfixes
3.0.1 (2019-10-09)
- Refactored with go mod support
- Added ability to comment lines
- Only one host per line for easier management
2.1.0 (2015-06-08)
- Added Windows support.
- Added command-line docs.
2.0.0 (2015-05-04)
- Breaking API change.
- Add support for adding and removing multiple hosts.
- Added
--all
flag.
- Handle malformed IP addresses.
1.0.0 (2015-05-03)
License
MIT
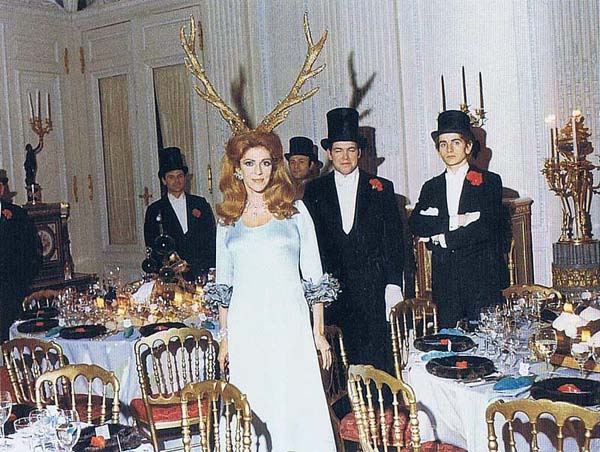