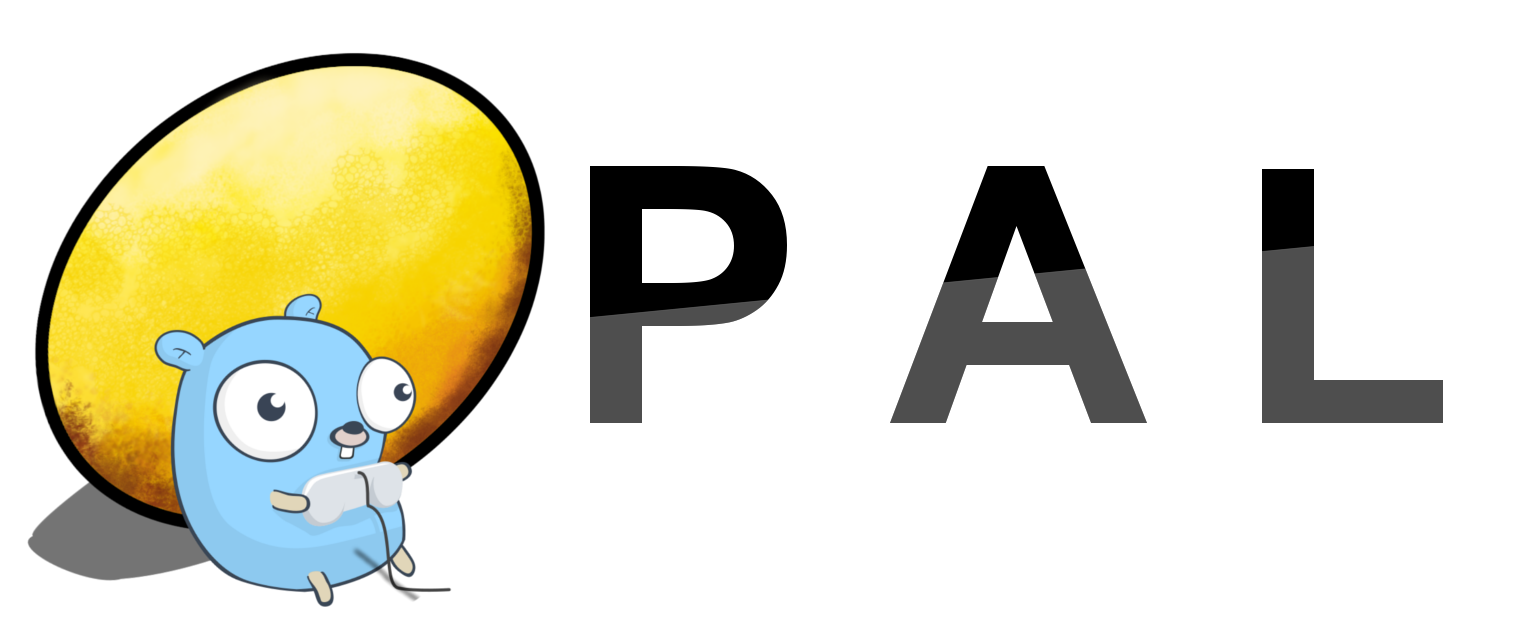
Opal - A HTTP2 Webframework in Go

Opal is a simple HTTP2 web-framework implemented in Go (Golang), made for fast and reliable development. Opal is a powerful package for quickly writing modular web applications/services in Go.
Content
- Installation
- Examples
- Implementations
- Todo
- Dependencies
- Tests
- Documentation
- Authors
Installation
To use Opal, you need to install Go and set your Go workspace first, see Go installation docs.
- Download and install it:
$ go get -u github.com/SveinungOverland/opal
- Import it in your code:
import (
"github.com/SveinungOverland/opal"
"github.com/SveinungOverland/opal/http"
"github.com/SveinungOverland/opal/router"
)
Examples
Basic Usage
srv, err := opal.NewTLSServer("./server.crt", "./server.key")
r := router.NewRouter("/")
// A simple GET-endpoint
r.Get("/", func(req *http.Request, res *http.Response) {
res.String(200, "Hello World! :D")
})
// A simple PUT-endpoint
r.Put("/:id", func(req *http.Request, res *http.Response) {
id := req.Param("id") // Read path parameter
res.String(200, id)
})
srv.Register(r) // Register router
srv.Listen(443)
Server Push
srv, err := opal.NewTLSServer("./server.crt", "./server.key")
r := router.NewRouter("/")
// A simple endpoint that returns a html document
r.Get("/", func(req *http.Request, res *http.Response) {
res.HTML("./index.html", nil)
res.Push("/static/app.js") // Push app.js
res.Push("/static/index.css") // Push index.css
})
// Register all files in given path as accessible files,
// without the need to register every single file
r.Static("/static", "./MY_STATIC_PATH")
srv.Register(r)
srv.Listen(443)
Middlewares
// Authorization middleware
auth := func(req *http.Request, res *http.Response) {
token := req.Query("token")
if token != "MY_SECRET_PASSWORD" {
res.Unauthorized()
req.Finish()
// Finish tells Opal that the request ends here and doesn't
// get passed along to other endpoint handlers or middleware
}
}
srv, err := opal.NewTLSServer("./server.crt", "./server.key")
srv.Use(corsHandler) // Adding an optional cors middleware
r := router.NewRouter("/")
// \/ Auth middleware function that is defined above gets added here
r.Post("/todo", auth, func(req *http.Request, res *http.Response) {
task := string(req.Body)
// Send new todo item
res.JSON(201, http.JSON {
"todo": task,
"done": false,
})
})
srv.Register(r)
srv.Listen(443)
Static Content
Opal provides an easy way to serve static content, like html, css and js files generated by for example a React or Vue build and is easily achieved by providing a path to the files to router.Static()
srv, err := opal.NewTLSServer("./server.crt", "./server.key")
r := router.NewRouter("/")
r.Static("/", "./build") // Serves the entire build folder on root path
srv.Register(r)
srv.Listen(443)
Implementations
Opal implements a robust HTTP2-library managing multiple clients with REST-support, Server-Push, and support for serving static files.
Core of the HTTP/2 Protocol
Implemented most of the HTTP2-protocol, specified by RFC7540
Created a robust and solid HPACK library, RFC7541
HTTP Router library
A high preformance HTTP-Router with parameter- and filehandling-functionality.
Todo
- Add support for HTTP/1.1
- Implement Stream Priority, RFC7540 Section 5.3
- Implement better support for middlewares
- Implement server push for static routes
Dependencies
- crypto/tls - A TLS-library from the standard-library. Docs
- github.com/go-test/deep - A library for test-support. Github Repo
- github.com/fatih/color - A color-library for changing colors in the console. Github Repo
Tests
For running the test the following command can be executed at the root directory.
go test -v ./...
For seeing test-coverage the following commands can be exectuted:
go test -v ./... -coverageprofile=coverage.out
go tool cover -html=coverage.out
Documentation
GoDoc is generated and hosted at godoc.org/opal.
At the bottom of the page there is also docs for the subpackages (like http and hpack)
HTTP Docs (Request and Response)
GoDoc: https://godoc.org/github.com/SveinungOverland/opal/http
Router Docs (Get, Post, Put...)
GoDoc: https://godoc.org/github.com/SveinungOverland/opal/router
HPACK Docs
GoDoc: https://godoc.org/github.com/SveinungOverland/opal/hpack
Authors
