go-cli
A simple CLI library written in Go.
The library is intended to be used for a whole application, meaning only one CLI is possible for the whole app.
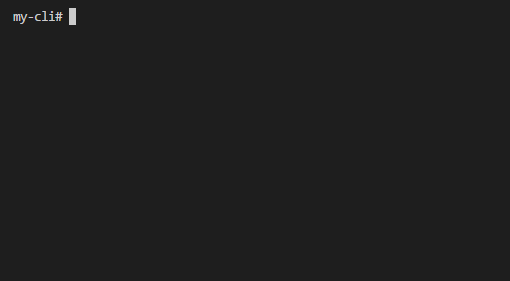
Features
- Command autocompletion
- Bash-like command history
- Easy to use!
Usage
import "github.com/alexrsagen/go-cli"
Get started by taking a look at the example usage in Exec.
CommandList
type CommandList map[string]*Command
This is a collection of commands stored by name.
Example command list:
var list cli.CommandList
list = cli.CommandList{
"command": &cli.Command{
Description: "Example command",
Handler: func(args []string) {
cli.Println("Example command ran!")
},
},
"submenu": &cli.Command{
Description: "A nested menu of commands",
Handler: func(args []string) {
cli.SetList(list["submenu"].List)
cli.SetPrefix("my-cli(submenu)# ")
},
List: cli.CommandList{
"command": &cli.Command{
Description: "Example command",
Handler: func(args []string) {
cli.Println("Submenu example command ran!")
},
},
"return": &cli.Command{
Description: "Return from submenu context",
Handler: func(args []string) {
cli.SetList(list)
cli.SetPrefix("my-cli# ")
},
},
},
},
"another_submenu": &cli.Command{
Description: "Another nested menu of commands",
Handler: func(args []string) {
cli.SetList(list["submenu"].List)
cli.SetPrefix("my-cli(submenu)# ")
},
List: cli.CommandList{
"command": &cli.Command{
Description: "Example command",
Handler: func(args []string) {
cli.Println("Another submenu example command ran!")
},
},
"return": &cli.Command{
Description: "Return from submenu context",
Handler: func(args []string) {
cli.SetList(list)
cli.SetPrefix("my-cli# ")
},
},
},
},
}
CommandHandler
type CommandHandler func(args []string)
This defines the prototype for a function ran when executing a Command
Command
type Command struct {
Description string
Arguments []string
Handler CommandHandler
List CommandList
}
This is a structure for storing a single command item. You cannot store the name of a command inside itself. Use a CommandList to store commands by name.
A Command containing other commands may not have a handler set. If you do this, it will result in a runtime panic.
Example command item:
var item *cli.Command
item = &cli.Command{
Description: "Example command",
Handler: func(args []string) {
cli.Println("Example command ran!")
},
}
Exec
func Exec(path []string) bool
This function attempts to execute a single command, and returns true if the command executed.
Example usage:
import "os"
import "github.com/alexrsagen/go-cli"
func main() {
cli.SetList(cli.CommandList{
"command": &cli.Command{
Description: "Example command",
Handler: func(args []string) {
cli.Println("Example command ran!")
},
}
})
// Default prefix is "# ", but you can change it like so:
// cli.SetPrefix("my-cli# ")
// Executes a command directly, if one is given in arguments.
// Otherwise creates a CLI.
if len(os.Args) > 1 {
if !cli.Exec(os.Args[1:]) {
os.Exit(1)
}
} else {
err := cli.Run()
if err != nil {
panic(err) // Received an error event from termbox
}
}
}
Field
type Field struct {
DisplayName, Input string
Mask rune
Format *regexp.Regexp
}
This is a structure containing a single form field.
FieldCategory
type FieldCategory struct {
DisplayName string
Fields FieldList
}
This is a FieldList with a title.
FieldList
type FieldList []*Field
This is a collection of form fields.
FieldCategoryList
type FieldCategoryList []*FieldCategory
This is a collection of field categories.
func (fl FieldList) Form() bool
func (fcl FieldCategoryList) Form() bool
These functions render a series of input fields to be filled before returning. Should be used within a CommandHandler. The FieldCategoryList Form()
function also renders its category titles.
The return value is false
if the input was cancelled or if inputs did not validate, otherwise true
.
Example usage:
func myHandler(args []string) {
field1 := &cli.Field{DisplayName: "Field 1"}
field2 := &cli.Field{DisplayName: "Field 2"}
field3 := &cli.Field{DisplayName: "Field 3"}
field4 := &cli.Field{DisplayName: "Field 4"}
field5 := &cli.Field{DisplayName: "Field 5"}
field6 := &cli.Field{DisplayName: "Field 6"}
fcl := cli.FieldCategoryList{
&cli.FieldCategory{
DisplayName: "Category 1",
Fields: cli.FieldList{
field1,
field2,
field3,
},
},
&cli.FieldCategory{
DisplayName: "Category 2",
Fields: cli.FieldList{
field4,
field5,
field6,
},
},
}
fcl.Form()
cli.Println("Form filled!\n")
cli.Printf("%s: %s\n", field1.DisplayName, field1.Input)
cli.Printf("%s: %s\n", field2.DisplayName, field2.Input)
cli.Printf("%s: %s\n", field3.DisplayName, field3.Input)
cli.Printf("%s: %s\n", field4.DisplayName, field4.Input)
cli.Printf("%s: %s\n", field5.DisplayName, field5.Input)
cli.Printf("%s: %s\n", field6.DisplayName, field6.Input)
}
SetPrefix
func SetPrefix(s string)
This function sets the CLI input prefix string.
Example usage: see Exec
SetList
func SetList(l CommandList)
This function sets the CLI command list.
Example usage: see Exec
Run
func Run() error
This function sets up a new CLI on the process tty.
Example usage: see Exec
Printf
func Printf(format string, a ...interface{})
A wrapper around fmt.Sprintf.
The point of the wrapper function is to be able to correctly write the output to the terminal created by termbox. Falls back to calling fmt.Printf directly when a terminal is has not been started.
Println
func Println(a ...interface{})
A wrapper around fmt.Sprintln.
The point of the wrapper function is to be able to correctly write the output to the terminal created by termbox. Falls back to calling fmt.Println directly when a terminal is has not been started.