image-slicer
Split images into tiles.
Perform advanced image processing in Go - slice images into tiles, combine the tiles.

Idea and Inspiration
I was tasked with building a puzzle game in Go. I struggled to find an article or a library that can accomplish image slicing.
Eventually, I had to settle down with python's image-slicer, and build a microservice
Game-Engine.
This incident made me realize that I can build a 35x faster library in Go. Hence, the inception of image-slicer.
Usecase 1: Split images into tiles
Grid [2*2]
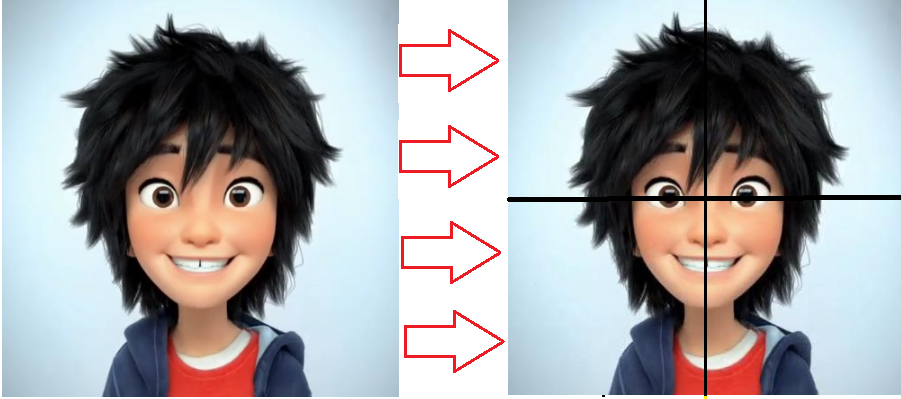
Grid [3*3]
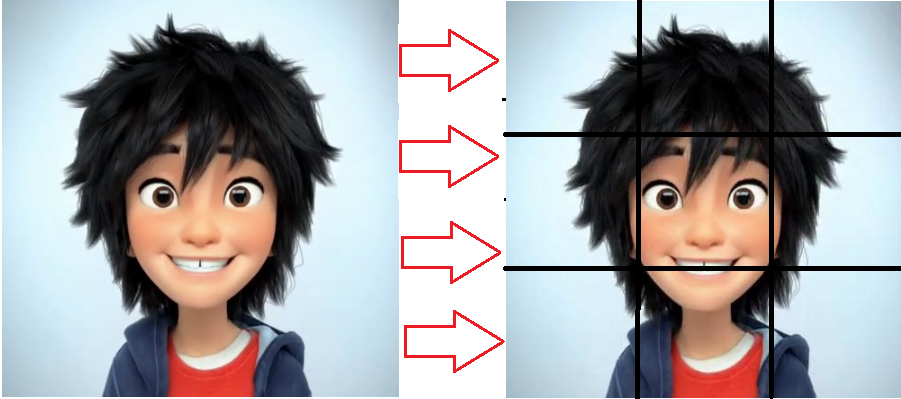
Grid [4*4]
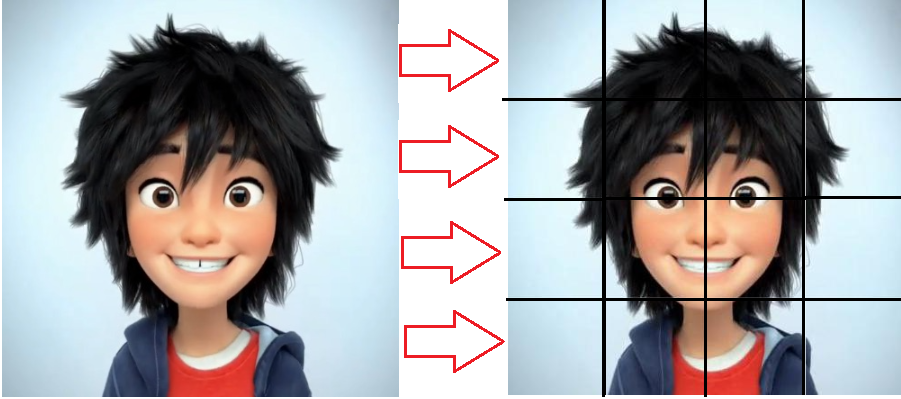
Usecases 2: Join the tiles back together
Grid [2*2]
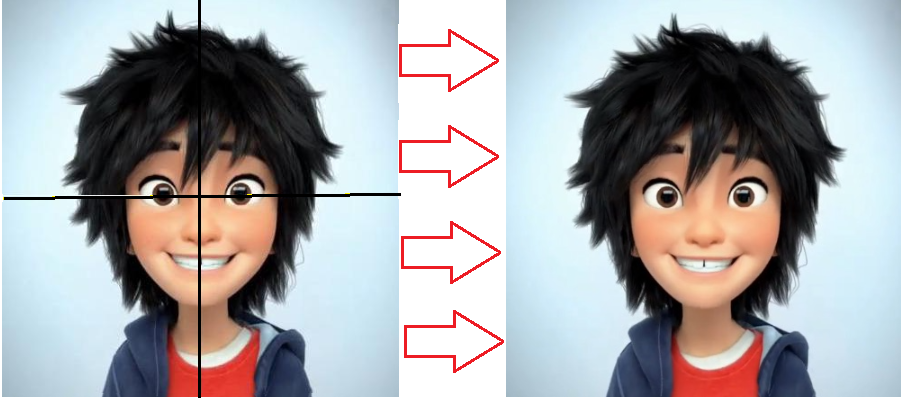
Grid [3*3]
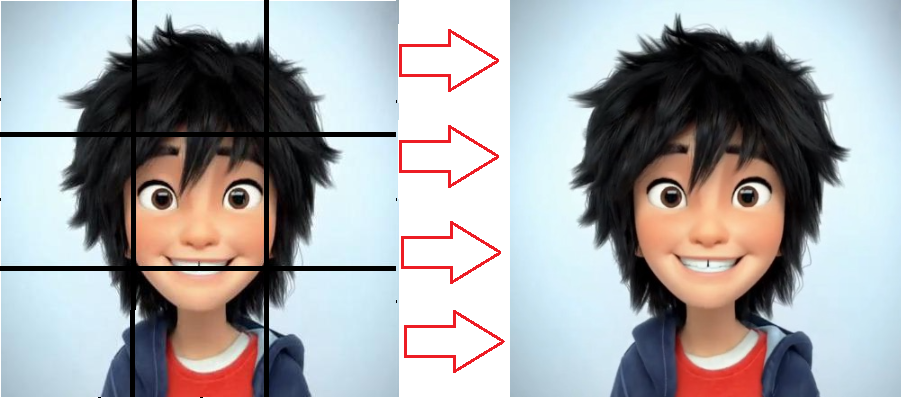
Grid [4*4]
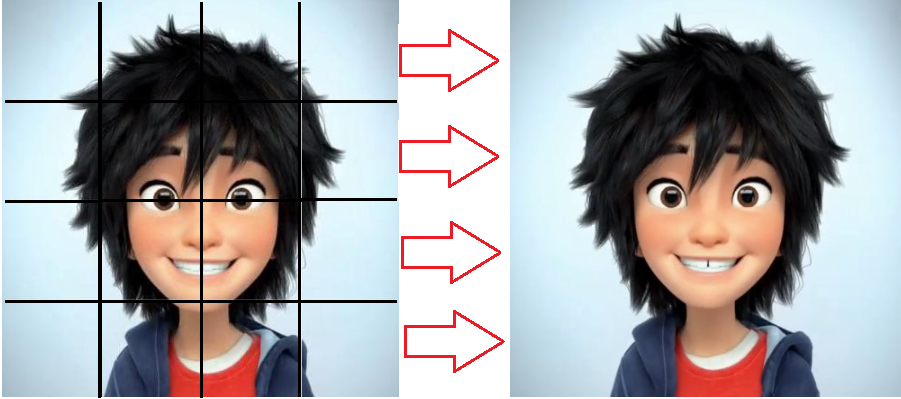
Support
You can file an Issue.
See documentation in Go.Dev
Getting Started
Download
go get -u github.com/goferHiro/image-slicer
Example
Slice Images
Click to Expand
imgUrl := "https://static.wikia.nocookie.net/big-hero-6-fanon/images/0/0f/Hiro.jpg/revision/latest?cb=20180511180437"
img := imageslicer.GetImageFromUrl(imgUrl)
if img == nil {
log.Fatalln("invalid image url or image format not supported!")
}
grid := [2]uint{2, 2} //rows,columns
tiles := imageslicer.Slice(img, grid)
expectedTiles := int(grid[0] * grid[1])
if len(tiles) != expectedTiles {
log.Fatalf("expected %d rcvd %d\n", expectedTiles, len(tiles))
}
Join the tiles back
Click to Expand
//lets join the tiles back
joinedImg, err := imageslicer.Join(tiles, grid)
if err != nil {
log.Fatalf("joining tiles failed due to %s", err)
}
shapeJ := joinedImg.Bounds()
shapeI := img.Bounds()
fmt.Println(shapeJ, shapeI) //shape might change due to pixel loss
}
Get Images
//From base64
base64str := "" //very long.
img, err := imageslicer.GetImageFromBase64(base64str)
//From urls
imgUrl := "https://static.wikia.nocookie.net/big-hero-6-fanon/images/0/0f/Hiro.jpg/revision/latest?cb=20180511180437"
img := imageslicer.GetImageFromUrl(imgUrl)
Inspired from ...
Important Notes/Caveats/Pitfalls
- Use the latest release.
Slice(img,grid)
- ensure grid[0]*grid[1]>0.
- ensure img is not nil.
- its best if the img is downsized to the area of grid to avoid pixel losses. //Will bring support in future releases
Join(tiles,grid)
- ensure that the grid is the same grid used originally for slicing.
- the grid is utilized to figure out positions of tiles & the dimensions of the final image.
- For best results, ensure the tiles were generated by image-slicer.
GetImageFromBase64(base64str)
- Supports the image tag format i.e "data:image/jpeg;base64,/" as well
- Supports jpeg,png only
- Does not support svg, or any other formats
GetImageFromUrl(imgUrl)
- Expects jpeg,png formats only
Contribution
- You can submit an issue or create a Pull Request (PR)