take

Simple RethinkDB orm-like wrapper based on gorethink
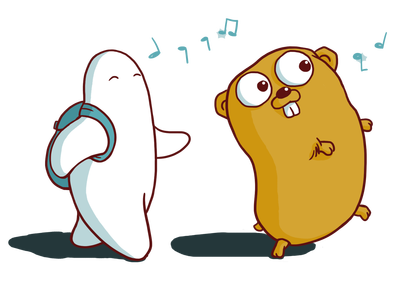
Installation
go get github.com/loeffel-io/take
Components
Component: Connect
Basic Connection
package main
import (
"os"
r "gopkg.in/gorethink/gorethink.v4"
"github.com/loeffel-io/take"
)
var databaseSession *r.Session
func init(){
// Init database
databaseSession = take.Connect(take.Connection{
Address: os.Getenv("RETHINKDB_ADDRESS") + ":" + os.Getenv("RETHINKDB_PORT"),
Database: os.Getenv("RETHINKDB_DATABASE"),
Username: os.Getenv("RETHINKDB_USERNAME"),
Password: os.Getenv("RETHINKDB_PASSWORD"),
})
}
Connection Pool
package main
import (
"os"
r "gopkg.in/gorethink/gorethink.v4"
"github.com/loeffel-io/take"
)
var databaseSession *r.Session
func init(){
// Init database
databaseSession = take.Connect(take.Connection{
Address: os.Getenv("RETHINKDB_ADDRESS") + ":" + os.Getenv("RETHINKDB_PORT"),
Database: os.Getenv("RETHINKDB_DATABASE"),
Username: os.Getenv("RETHINKDB_USERNAME"),
Password: os.Getenv("RETHINKDB_PASSWORD"),
InitialCap: 10,
MaxOpen: 10,
})
}
Secure Connection
e.g.: compose.io
package main
import (
"os"
r "gopkg.in/gorethink/gorethink.v4"
"github.com/loeffel-io/take"
)
var databaseSession *r.Session
func init(){
// Init database
databaseSession = take.Connect(take.Connection{
Address: os.Getenv("RETHINKDB_ADDRESS") + ":" + os.Getenv("RETHINKDB_PORT"),
Database: os.Getenv("RETHINKDB_DATABASE"),
Username: os.Getenv("RETHINKDB_USERNAME"),
Password: os.Getenv("RETHINKDB_PASSWORD"),
AuthKey: os.Getenv("RETHINKDB_AUTHKEY"),
CertPath: os.Getenv("RETHINKDB_CERT_PATH"),
})
}
Component: Database
Create Database
Create database if not exists
package main
import (
r "gopkg.in/gorethink/gorethink.v4"
"github.com/loeffel-io/take"
)
var databaseSession *r.Session
func main(){
take.DatabaseCreate("test", databaseSession)
}
Check if Database exists
package main
import (
"log"
r "gopkg.in/gorethink/gorethink.v4"
"github.com/loeffel-io/take"
)
var databaseSession *r.Session
func main(){
exists := take.DatabaseExists("test", databaseSession)
if exists{
log.Println("Database exists")
return
}
log.Println("Database not exists")
}
Component: Index
Create Index
Create index if not exists
package main
import (
r "gopkg.in/gorethink/gorethink.v4"
"github.com/loeffel-io/take"
)
var databaseSession *r.Session
func main(){
take.IndexCreate("table", "index", "field", databaseSession)
}
Check if Index exists
package main
import (
"log"
r "gopkg.in/gorethink/gorethink.v4"
"github.com/loeffel-io/take"
)
var databaseSession *r.Session
func main(){
exists := take.IndexExists("table", "index", databaseSession)
if exists{
log.Println("Index exists")
return
}
log.Println("Index not exists")
}
Component: Table
Create Tables
Create tables if not exists
package main
import (
r "gopkg.in/gorethink/gorethink.v4"
"github.com/loeffel-io/take"
)
var databaseSession *r.Session
func main(){
take.TablesCreate([]string{"table1", "table2"}, databaseSession)
}
Check if Table exists
package main
import (
"log"
r "gopkg.in/gorethink/gorethink.v4"
"github.com/loeffel-io/take"
)
var databaseSession *r.Session
func main(){
exists := take.TableExists("table", databaseSession)
if exists{
log.Println("Table exists")
return
}
log.Println("Table not exists")
}
Component: User
Setup user
Create or update user and set user permissions for the current database
package main
import (
r "gopkg.in/gorethink/gorethink.v4"
"github.com/loeffel-io/take"
)
var databaseSession *r.Session
func main(){
user := take.User{
ID: "username",
Password: "password",
Permissions: take.UserPermissions{
Read: true,
Write: false,
Config: false,
},
}
take.SetupUser(user, databaseSession)
}
Component: Insert
Insert
Insert single object
package main
import (
r "gopkg.in/gorethink/gorethink.v4"
"github.com/loeffel-io/take"
)
var databaseSession *r.Session
type Task struct{
ID int `gorethink:"id"`
Name string `gorethink:"name"`
}
func main(){
firstTask := Task{
ID: 1,
Name: "First Task",
}
take.Insert("table", firstTask, databaseSession)
}
InsertMany
Insert multiple objects
package main
import (
r "gopkg.in/gorethink/gorethink.v4"
"github.com/loeffel-io/take"
)
var databaseSession *r.Session
type Task struct{
ID int `gorethink:"id"`
Name string `gorethink:"name"`
}
func main(){
firstTask := Task{
ID: 1,
Name: "First Task",
}
secondTask := Task{
ID: 2,
Name: "Second Task",
}
take.Insert("table", []interface{}{firstTask, secondTask}, databaseSession)
}
InsertOrUpdate
Insert single object or update object if exists
package main
import (
r "gopkg.in/gorethink/gorethink.v4"
"github.com/loeffel-io/take"
)
var databaseSession *r.Session
type Task struct{
ID int `gorethink:"id"`
Name string `gorethink:"name"`
}
func main(){
firstTask := Task{
ID: 1,
Name: "First Task",
}
take.InsertOrUpdate("table", firstTask, databaseSession)
}
InsertOrUpdateMany
Insert multiple object or update objects if exists
package main
import (
r "gopkg.in/gorethink/gorethink.v4"
"github.com/loeffel-io/take"
)
var databaseSession *r.Session
type Task struct{
ID int `gorethink:"id"`
Name string `gorethink:"name"`
}
func main(){
firstTask := Task{
ID: 1,
Name: "First Task",
}
secondTask := Task{
ID: 2,
Name: "Second Task",
}
take.InsertOrUpdateMany("table", []interface{}{firstTask, secondTask}, databaseSession)
}
Component: Update
Update
Update single object by id
package main
import (
r "gopkg.in/gorethink/gorethink.v4"
"github.com/loeffel-io/take"
)
var databaseSession *r.Session
type Task struct{
ID int `gorethink:"id"`
Name string `gorethink:"name"`
}
func main(){
firstTask := Task{
ID: 1,
Name: "First Task updated",
}
take.Update("table", firstTask.ID, firstTask, databaseSession)
}
Component: Delete
Delete
Delete single object by id
package main
import (
r "gopkg.in/gorethink/gorethink.v4"
"github.com/loeffel-io/take"
)
var databaseSession *r.Session
func main(){
take.Delete("table", "id", databaseSession)
}
Sentry support
This package supports sentry.io real time error reporting.
More informations: Sentry golang docs
package main
import "github.com/getsentry/raven-go"
func init() {
// Setup sentry
raven.SetDSN("https://<key>:<secret>@sentry.io/<project>")
// Setup cronjobs ...
}